Twitter OAuth 2 Authentication using NextAuth in Next.js
In this tutorial, we will learn how to sign in users using Twitter’s new OAuth 2. Twitter is a popular social media platform and NextAuth is an authentication library for Next.js which handles all the complex layers for authentication. It supports a wide range of authentication methods like Google, Github, LinkedIn, Twitter, Email, etc. We will use TwitterProvider for our use-case.
Prerequisites
- Node.js
- Basic understanding of Next.js
Create Next app
Start by creating a Next.js app.
npx create-next-app@latest
Provide the necessary details to initialize the app.
App-name: I will just put my-app.
TypeScript: I won’t be using TypeScript with this project so I am going to select No in the second option.
ESlint: I will be using ESlint for the project so that will be a yes.
Tailwind: We won’t really need styling in this tutorial, so I am going to opt out of using Tailwind and save some space.
src?: I like to put everything on my ‘src’ so that will be a yes too.
After the installation is complete, head over to your app folder.
Install NextAuth
Now we will install next-auth which handles most of the authentication process.
npm i --save next-auth
Now think of the structure of your app. Users will visit a certain page where they will be asked to log in if they are not signed in. They will click on sign in and will be brought to a page showing Twitter Sign In as an option.
In the pages folder, create a folder called api and another called auth inside api and create a file inside auth and name it [...nextauth].js
like so:
- pages
- api
- auth
- […nextauth].js
- auth
- api
The three dots defines catch-all routes. It means all routes in the form api/auth/ is handled by the same file i.e [...nextauth].js
. So api/auth/abc or api/auth/abc/def is always caught by the [...nextauth].js
.
In the [...nextauth.js]
file add the following code:
import NextAuth from "next-auth";
import TwitterProvider from "next-auth/providers/twitter";
export const authOptions = {
providers: [
TwitterProvider({
clientId: process.env.TWITTER_CLIENT_ID,
clientSecret: process.env.TWITTER_CLIENT_SECRET,
version: "2.0",
}),
],
};
export default NextAuth(authOptions);
We are using TwitterProvider from NextAuth which will handle all the Twitter-related authentication. The provider needs clientId
and clientSectret
which we need to get from Twitter. The best practice is to put clientId
and clientSectret
in an env file. We will now see how to get clientId
and clientSecret
from Twitter.
Get Client Secrets From Twitter
Head over to Twitter Developer Page and sign up for a developer account. Choose the free signup option for this tutorial. After signing up, you are redirected to the developer portal.
On the left side of the dashboard, choose projects and apps and select your app like in the image.
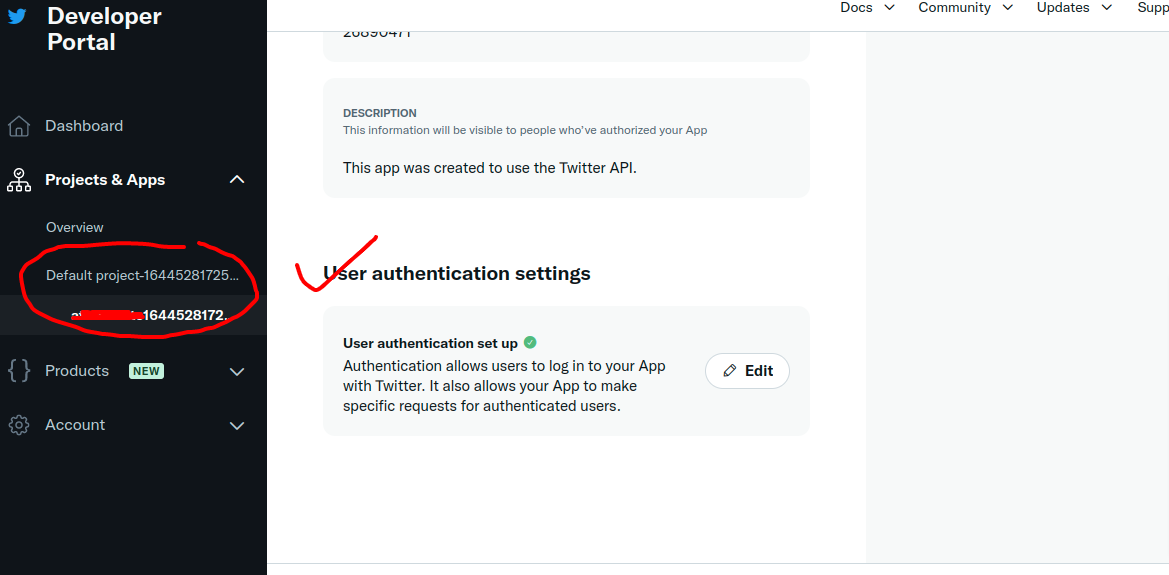
Once you click on it, scroll below and you should see a setting called “User authentication settings”. I have already configured my but it is easy to do so.
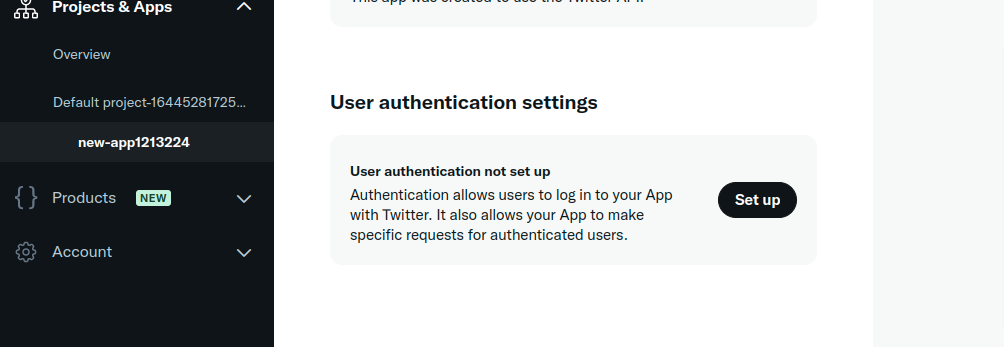
Here is an image of what my settings look like.
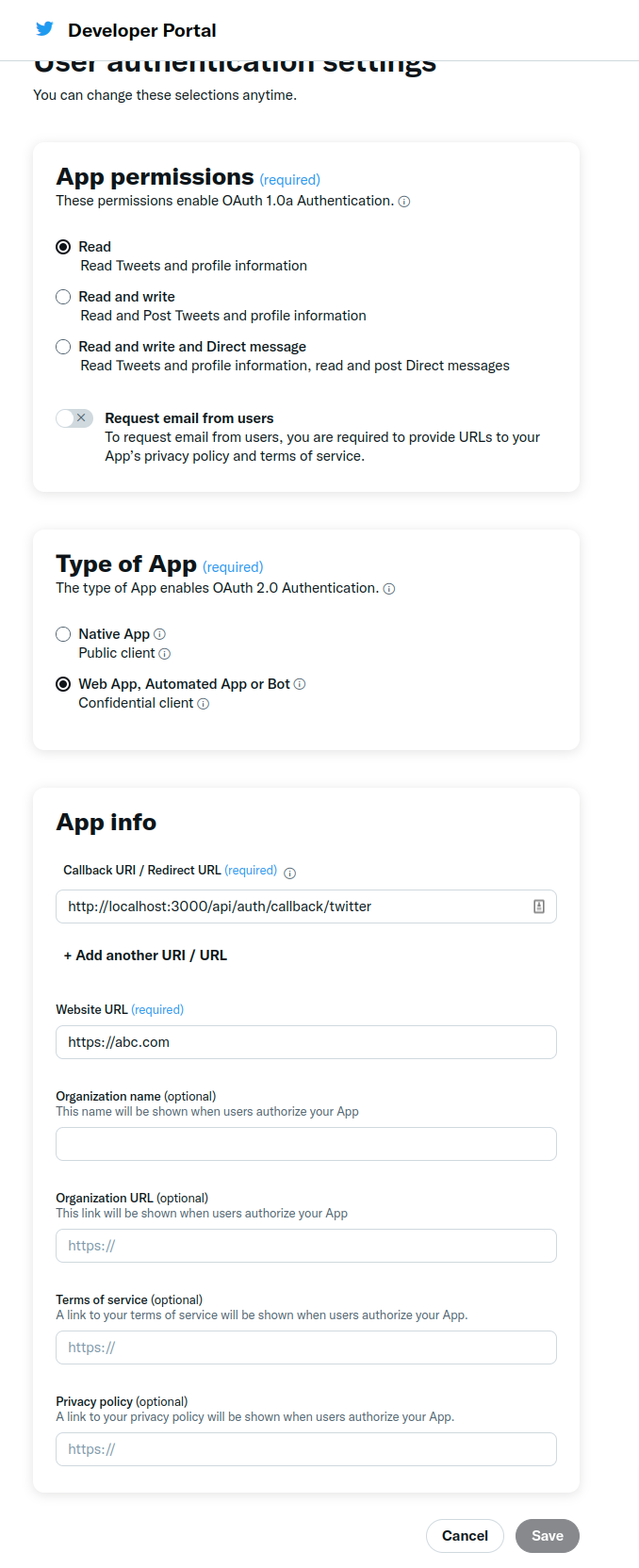
For app permissions, I am just going to select Read. Type of app is webapp. The important part in App Info is callback URI which should be http://localhost:port/api/auth/callback/twitter. In my case, my Next app is running at port 3000 so I used 3000.
You don’t need to create the callback URI. Remember, our catch-all route that was api/auth/[…nextauth.js] handles api/auth/callback/twitter as well.
For testing, you can put any random website( make sure it’s HTTPS) but for production, you need to add your actual website. Also, terms of service and privacy policy links are very essential for production as they affect your app verification process.
After saving you will get your client ID and secret like so.
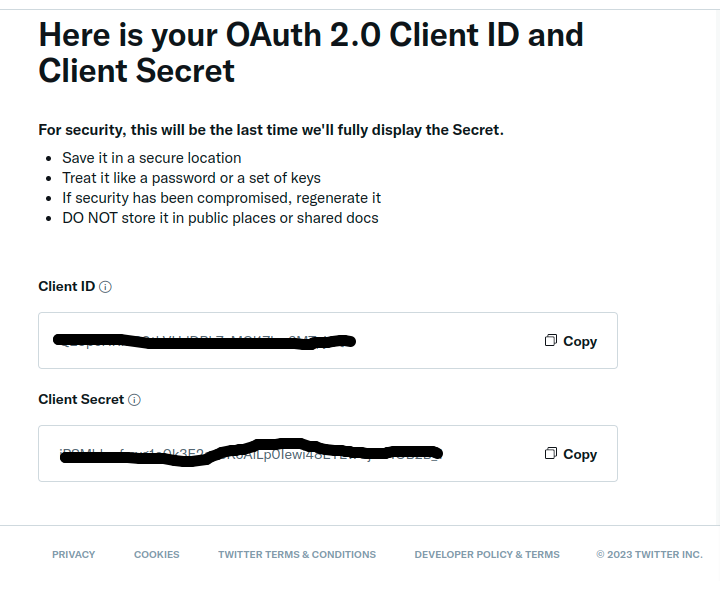
Create a folder named env in the root of the project and another file named .env
. Copy your clientId and secret and paste it accordingly.
TWITTER_CLIENT_ID = your_client_id;
TWITTER_CLIENT_SECRET = your_client_secret;
Authentication Process
Now go to your _app.js
file and add replace the code with the following code.
import { SessionProvider } from "next-auth/react";
export default function App({
Component,
pageProps: { session, ...pageProps },
}) {
return (
<SessionProvider session={session}>
<Component {...pageProps} />
</SessionProvider>
);
}
Here, we are using SessionProvider from NextAuth which basically makes session properties available to all components it wraps. We are passing session as props as well.
In the index.js
file inside pages,
we will check if the user is signed in or not and if they are signed in we will give the option to sign out and vice versa.
Our index.js
page:
import { signIn, signOut, useSession } from "next-auth/react";
import { useEffect } from "react";
export default function Home() {
const { data: session } = useSession();
useEffect(() => {
console.log("session object is", session);
}, []);
return (
<>
{!session && (
<>
Not logged in <br />
<button onClick={() => signIn()}>Log In</button>
</>
)}
//Once user is logged in, check if session is present and do cool stuffs
{session && (
<>
Logged in as {session.user.name} <br />
<button onClick={() => signOut()}>Log out</button>
</>
)}
</>
);
}
We are importing signIn, signOut
and useSession
from next-auth. signIn
and signOut
perform the operation according to their names and useSession gives us information about the session once we are logged in. You can log it to console and view it like I have shown.
Now, go to your Next.js app starting page(eg: http://localhost:3000) and click on Login and you will be redirected to a page(/api/auth/signin) that shows the Twitter Login option. Now login in using your Twitter account and you will be redirected back to the homepage (index.js
) as an authenticated user and you can view the session object in the console. Thus, we have accomplished Twitter Authentication process using NextAuth in our Next.js app.
If you want more tutorials like this or have questions about this tutorial or Next.js in general, follow me on Twitter. Also if you want to receive it in your email, subscribe to the newsletter! Thanks for your time!